Download Garfield Comic From the Command Line
1 min read • 132 words
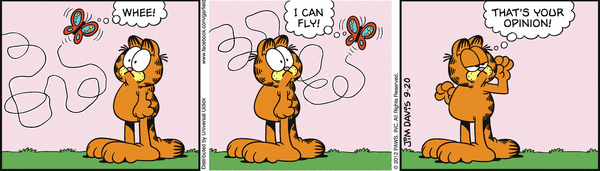
above: © Whoever owns the copyrights for the Garfield comics
Garfield has always been one of my favorite comic strips. I read it every day, and always save the ones I like to a folder on my computer.
Because of my OCD, I insist on saving these files with their filenames as yyyy-mm-dd.jpg
. This way, Finder sorts them and I can easily revisit them in order.
To save myself some of the effort of renaming these files every day, I went ahead and wrote a ruby script that does it for me. I can get my daily dose of garfield simply by running this script and opening the file it drops on to my desktop.
Instructions below.
Save the following script to a file such as ~/Desktop/garfield.rb
and run it with ruby ~/Desktop/garfield.rb 2012-09-27
or just ruby ~/Desktop/garfield.rb
.
require 'net/http'
require 'uri'
require "open-uri"
# This regular expression matches a date that
# is in the form ####-##-##
regex = /\d\d\d\d-\d\d-\d\d/
# Get the date for the comic we are retreiving,
# what's passed to the command line or the current
# date if nothing
if(ARGV[0])
date = ARGV[0].gsub(/-/, "\/")
else
# Match the date in time.now.to_s using the regex,
# and then replace the dashes with slashes for the url
date = regex.match(Time.now.to_s).to_s.gsub(/-/, "\/")
end
url = "http://www.gocomics.com/garfield/#{date}"
# Perform the HTTP get request
url = URI.parse(url)
req = Net::HTTP::Get.new(url.path)
res = Net::HTTP.start(url.host, url.port) { |http|
http.request(req)
}
# Pull the src url for the comic image itself from
# the html for the page
url = /img alt="Garfield" .*src="([a-z:\/.0-9]*).*/.match(res.body)[1]
# Reformat the date to contain '-'es instead of '/'
# es to save it to the desktop
date = date.gsub(/\//, "-")
# Save the file to the Desktop in the format yyyy-mm-dd.gif
open(url) {|f|
File.open(File.expand_path("~/Desktop/#{date}.gif"),"wb") do |file|
file.puts f.read
end
}